How To Draw A Image In Java
Images
last modified July 13, 2020
In this part of the Java 2nd tutorial, we will work with images.
BufferedImage
is a fundamental class for working with images in Java 2D. Information technology is a rectangle of pixels stored in retentivity.
Displaying an Image
In the first case, nosotros display an image on the console.
com/zetcode/DisplayImageEx.java
package com.zetcode; import java.awt.Dimension; import java.awt.EventQueue; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Prototype; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JPanel; class Surface extends JPanel { individual Image mshi; public Surface() { loadImage(); setSurfaceSize(); } individual void loadImage() { mshi = new ImageIcon("mushrooms.jpg").getImage(); } private void setSurfaceSize() { Dimension d = new Dimension(); d.width = mshi.getWidth(zip); d.elevation = mshi.getHeight(nix); setPreferredSize(d); } private void doDrawing(Graphics g) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(mshi, 0, 0, null); } @Override public void paintComponent(Graphics g) { super.paintComponent(1000); doDrawing(k); } } public grade DisplayImageEx extends JFrame { public DisplayImageEx() { initUI(); } private void initUI() { add(new Surface()); pack(); setTitle("Mushrooms"); setLocationRelativeTo(nix); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void primary(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { DisplayImageEx ex = new DisplayImageEx(); ex.setVisible(truthful); } }); } }
In the example, we display an image on the panel. The window is resized to fit the size of the image.
private void loadImage() { mshi = new ImageIcon("mushrooms.jpg").getImage(); }
We use the ImageIcon
form to load the epitome. The prototype is located in the current working directory.
private void setSurfaceSize() { Dimension d = new Dimension(); d.width = mshi.getWidth(null); d.elevation = mshi.getHeight(null); setPreferredSize(d); }
Nosotros make up one's mind the size of the loaded image. With the setPreferredSize()
method, we set the preferred size of the Surface
console. The pack()
method of the JFrame
container will cause the frame to fit the size of its children. In our case the Surface
panel. As a upshot, the window volition be sized to exactly display the loaded image.
private void doDrawing(Graphics thou) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(mshi, 0, 0, aught); }
The image is fatigued on the panel using the drawImage()
method. The last parameter is the ImageObserver
class. It is sometimes used for asynchronous loading. When nosotros do not need asynchronous loading of our images, nosotros tin can just put goose egg
at that place.
private void initUI() { ... pack(); ... }
The pack()
method sizes the container to fit the size of the child console.
Grayscale image
In computing, a grayscale digital prototype is an image in which the value of each pixel is a unmarried sample, that is, it carries the full (and only) information about its intensity. Images of this sort are composed exclusively of shades of neutral grayness, varying from black at the weakest intensity to white at the strongest. (Wikipedia)
In the adjacent example, we create a grayscale prototype with Coffee second.
com/zetcode/GrayScaleImage.java
package com.zetcode; import java.awt.Dimension; import coffee.awt.EventQueue; import java.awt.Graphics; import coffee.awt.Graphics2D; import java.awt.Image; import java.awt.image.BufferedImage; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JPanel; class Surface extends JPanel { private Image mshi; private BufferedImage bufimg; individual Dimension d; public Surface() { loadImage(); determineAndSetSize(); createGrayImage(); } private void determineAndSetSize() { d = new Dimension(); d.width = mshi.getWidth(null); d.top = mshi.getHeight(zip); setPreferredSize(d); } individual void createGrayImage() { bufimg = new BufferedImage(d.width, d.height, BufferedImage.TYPE_BYTE_GRAY); Graphics2D g2d = bufimg.createGraphics(); g2d.drawImage(mshi, 0, 0, null); g2d.dispose(); } private void loadImage() { mshi = new ImageIcon("mushrooms.jpg").getImage(); } private void doDrawing(Graphics g) { Graphics2D g2d = (Graphics2D) yard; g2d.drawImage(bufimg, zilch, 0, 0); } @Override public void paintComponent(Graphics thou) { super.paintComponent(grand); doDrawing(g); } } public class GrayScaleImageEx extends JFrame { public GrayScaleImageEx() { initUI(); } private void initUI() { Surface dpnl = new Surface(); add(dpnl); pack(); setTitle("GrayScale image"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(Cord[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { GrayScaleImageEx ex = new GrayScaleImageEx(); ex.setVisible(true); } }); } }
In that location are several ways to create a grayscale epitome. We exercise it by writing image data into buffered image of BufferedImage.TYPE_BYTE_GRAY
type.
bufimg = new BufferedImage(d.width, d.height, BufferedImage.TYPE_BYTE_GRAY);
Nosotros create a BufferedImage
grade of type BufferedImage.TYPE_BYTE_GRAY
.
Graphics2D g2d = bufimg.createGraphics(); g2d.drawImage(mshi, 0, 0, null);
Here nosotros draw the mushrooms image into the buffered prototype.
g2d.dispose();
Graphics objects created with createGraphics()
method should be manually released. Graphics objects which are provided equally arguments to the pigment()
and update()
methods of components are automatically released by the system when those methods render.
private void doDrawing(Graphics grand) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(bufimg, naught, 0, 0); }
The buffered image is drawn on the console with the drawImage()
method.
Flipped image
The following instance flips image. Nosotros are going to filter the image. At that place is a filter()
method which is transforming images.
com/zetcode/FlippedImageEx.java
bundle com.zetcode; import coffee.awt.Dimension; import java.awt.EventQueue; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Image; import java.awt.geom.AffineTransform; import java.awt.image.AffineTransformOp; import coffee.awt.epitome.BufferedImage; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JPanel; grade Surface extends JPanel { private Image mshi; private BufferedImage bufimg; private final int Space = 10; public Surface() { loadImage(); createFlippedImage(); setSurfaceSize(); } private void loadImage() { mshi = new ImageIcon("mushrooms.jpg").getImage(); } private void createFlippedImage() { bufimg = new BufferedImage(mshi.getWidth(null), mshi.getHeight(null), BufferedImage.TYPE_INT_RGB); Graphics gb = bufimg.getGraphics(); gb.drawImage(mshi, 0, 0, null); gb.dispose(); AffineTransform tx = AffineTransform.getScaleInstance(-1, 1); tx.interpret(-mshi.getWidth(null), 0); AffineTransformOp op = new AffineTransformOp(tx, AffineTransformOp.TYPE_NEAREST_NEIGHBOR); bufimg = op.filter(bufimg, nada); } private void setSurfaceSize() { int due west = bufimg.getWidth(); int h = bufimg.getHeight(); Dimension d = new Dimension(three*SPACE+2*westward, h+ii*Infinite); setPreferredSize(d); } private void doDrawing(Graphics yard) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(mshi, Infinite, Infinite, null); g2d.drawImage(bufimg, null, two*Space + bufimg.getWidth(), Space); } @Override public void paintComponent(Graphics k) { super.paintComponent(g); doDrawing(g); } } public class FlippedImageEx extends JFrame { public FlippedImageEx() { initUI(); } individual void initUI() { add together(new Surface()); pack(); setTitle("Flipped image"); setLocationRelativeTo(nothing); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void chief(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { FlippedImageEx ex = new FlippedImageEx(); ex.setVisible(true); } }); } }
In our code case, we horizontally flip an image.
AffineTransform tx = AffineTransform.getScaleInstance(-1, one); tx.translate(-castle.getWidth(naught), 0);
Flipping an image means scaling information technology and translating information technology. Then we practise an AffineTransform
operation.
AffineTransformOp op = new AffineTransformOp(tx, AffineTransformOp.TYPE_NEAREST_NEIGHBOR); bufferedImage = op.filter(bufferedImage, null)
This is 1 of the filtering operations available. This could be as well done past pixel manipulation. But Coffee 2d provides high level classes that make information technology easier to manipulate images. In our instance, the AffineTransformOp
grade performs scaling and translation on the prototype pixels.
private void doDrawing(Graphics thou) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(mshi, Infinite, SPACE, null); g2d.drawImage(bufimg, null, 2*Infinite + bufimg.getWidth(), SPACE); }
Both images are painted on the panel.
individual void setSurfaceSize() { int due west = bufimg.getWidth(); int h = bufimg.getHeight(); Dimension d = new Dimension(3*SPACE+2*w, h+2*Infinite); setPreferredSize(d); }
We set the preferred size for the panel. Nosotros calculate the size so that we can place 2 images on the panel and put some infinite between them, and the images and the window borders.
Blurred image
The next code case blurs an image. A mistiness means an unfocused image. To blur an epitome, we use a convolution operation. Information technology is a mathematical operation which is as well used in edge detection or noise emptying. Mistiness operations can be used in various graphical effects. For example creating speed illusion or showing an unfocused vision of a human.
The blur filter operation replaces each pixel in epitome with an boilerplate of the pixel and its neighbours. Convolutions are per-pixel operations. The same arithmetic is repeated for every pixel in the epitome. A kernel can be idea of equally a two-dimensional grid of numbers that passes over each pixel of an image in sequence, performing calculations along the mode. Since images can also be thought of as 2-dimensional grids of numbers, applying a kernel to an paradigm tin can exist visualized every bit a modest grid (the kernel) moving across a substantially larger grid (the image). (developer.apple tree.com)
com/zetcode/BlurredImageEx.java
package com.zetcode; import coffee.awt.Dimension; import java.awt.EventQueue; import coffee.awt.Graphics; import java.awt.Graphics2D; import java.awt.image.BufferedImage; import java.awt.image.BufferedImageOp; import java.awt.image.ConvolveOp; import java.awt.image.Kernel; import coffee.io.File; import java.io.IOException; import coffee.util.logging.Level; import java.util.logging.Logger; import javax.imageio.ImageIO; import javax.swing.JFrame; import javax.swing.JPanel; class Surface extends JPanel { private BufferedImage mshi; private BufferedImage bluri; public Surface() { loadImage(); createBlurredImage(); setSurfaceSize(); } private void loadImage() { endeavour { mshi = ImageIO.read(new File("mushrooms.jpg")); } catch (IOException ex) { Logger.getLogger(Surface.class.getName()).log( Level.WARNING, cypher, ex); } } private void createBlurredImage() { bladder[] blurKernel = { 1 / 9f, ane / 9f, one / 9f, 1 / 9f, 1 / 9f, i / 9f, i / 9f, one / 9f, 1 / 9f }; BufferedImageOp mistiness = new ConvolveOp(new Kernel(3, three, blurKernel)); bluri = mistiness.filter(mshi, new BufferedImage(mshi.getWidth(), mshi.getHeight(), mshi.getType())); } private void setSurfaceSize() { Dimension d = new Dimension(); d.width = mshi.getWidth(zero); d.height = mshi.getHeight(null); setPreferredSize(d); } individual void doDrawing(Graphics one thousand) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(bluri, cypher, 0, 0); } @Override public void paintComponent(Graphics 1000) { super.paintComponent(g); doDrawing(yard); } } public class BlurredImageEx extends JFrame { public BlurredImageEx() { setTitle("Blurred image"); add(new Surface()); pack(); setLocationRelativeTo(cipher); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void primary(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { BlurredImageEx ex = new BlurredImageEx(); ex.setVisible(true); } }); } }
In the code example, we load an epitome from the disk, perform a blur operation on the image, and display the effect on the window.
private void loadImage() { effort { mshi = ImageIO.read(new File("mushrooms.jpg")); } catch (IOException ex) { Logger.getLogger(Surface.class.getName()).log( Level.Alarm, null, ex); } }
The the read()
method of the ImageIO
class reads an paradigm from the disk and returns a BufferedImage
.
float[] blurKernel = { 1 / 9f, i / 9f, 1 / 9f, 1 / 9f, 1 / 9f, i / 9f, 1 / 9f, 1 / 9f, 1 / 9f };
This matrix is called a kernel. The values are weights that are applied to the neighbouring values of the pixel being changed.
BufferedImageOp blur = new ConvolveOp(new Kernel(3, 3, blurKernel)); bluri = mistiness.filter(mshi, new BufferedImage(mshi.getWidth(), mshi.getHeight(), mshi.getType()));
Here we use the mistiness filter on the epitome.
private void doDrawing(Graphics g) { Graphics2D g2d = (Graphics2D) g; g2d.drawImage(bluri, nada, 0, 0); }
The blurred image is fatigued on the window.
Reflection
In the side by side instance we testify a reflected epitome. This effect makes an illusion as if the epitome was reflected in h2o. The following code example was inspired by the code from jhlabs.com.
com/zetcode/ReflectionEx.java
packet com.zetcode; import java.awt.AlphaComposite; import java.awt.Colour; import java.awt.Dimension; import java.awt.EventQueue; import coffee.awt.GradientPaint; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.image.BufferedImage; import java.io.File; import java.util.logging.Level; import java.util.logging.Logger; import javax.imageio.ImageIO; import javax.swing.JFrame; import javax.swing.JPanel; class Surface extends JPanel { individual BufferedImage image; private BufferedImage refImage; individual int img_w; individual int img_h; private final int SPACE = 30; public Surface() { loadImage(); getImageSize(); createReflectedImage(); } private void loadImage() { try { image = ImageIO.read(new File("rotunda.jpg")); } catch (Exception ex) { Logger.getLogger(Surface.class.getName()).log( Level.WARNING, null, ex); } } private void getImageSize() { img_w = prototype.getWidth(); img_h = image.getHeight(); } individual void createReflectedImage() { float opacity = 0.4f; bladder fadeHeight = 0.3f; refImage = new BufferedImage(img_w, img_h, BufferedImage.TYPE_INT_ARGB); Graphics2D rg = refImage.createGraphics(); rg.drawImage(epitome, 0, 0, null); rg.setComposite(AlphaComposite.getInstance(AlphaComposite.DST_IN)); rg.setPaint(new GradientPaint(0, img_h * fadeHeight, new Color(0.0f, 0.0f, 0.0f, 0.0f), 0, img_h, new Color(0.0f, 0.0f, 0.0f, opacity))); rg.fillRect(0, 0, img_w, img_h); rg.dispose(); } private void doDrawing(Graphics chiliad) { Graphics2D g2d = (Graphics2D) m.create(); int win_w = getWidth(); int win_h = getHeight(); int gap = 20; g2d.setPaint(new GradientPaint(0, 0, Colour.black, 0, win_h, Colour.darkGray)); g2d.fillRect(0, 0, win_w, win_h); g2d.interpret((win_w - img_w) / 2, win_h / 2 - img_h); g2d.drawImage(epitome, 0, 0, nada); g2d.translate(0, 2 * img_h + gap); g2d.scale(1, -one); g2d.drawImage(refImage, 0, 0, zip); g2d.dispose(); } @Override public void paintComponent(Graphics g) { super.paintComponent(g); doDrawing(g); } @Override public Dimension getPreferredSize() { return new Dimension(img_w + 2 * Space, 2 * img_h + 3 * Space); } } public class ReflectionEx extends JFrame { public ReflectionEx() { initUI(); } individual void initUI() { add(new Surface()); pack(); setTitle("Reflection"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { ReflectionEx ex = new ReflectionEx(); ex.setVisible(true); } }); } }
In the example, we create an illusion of a reflected image.
refImage = new BufferedImage(img_w, img_h, BufferedImage.TYPE_INT_ARGB); Graphics2D rg = refImage.createGraphics(); rg.drawImage(paradigm, 0, 0, null);
A copy of a loaded image is created.
rg.setComposite(AlphaComposite.getInstance(AlphaComposite.DST_IN)); rg.setPaint(new GradientPaint(0, img_h * fadeHeight, new Color(0.0f, 0.0f, 0.0f, 0.0f), 0, img_h, new Colour(0.0f, 0.0f, 0.0f, opacity))); rg.fillRect(0, 0, img_w, img_h);
This is the nearly important part of the code. We make the second image transparent. But the transparency is non constant; the image gradually fades out. This is achieved with the GradientPaint
class.
g2d.setPaint(new GradientPaint(0, 0, Colour.black, 0, win_h, Color.darkGray)); g2d.fillRect(0, 0, win_w, win_h);
The groundwork of the window is filled with a gradient pigment. The paint is a smoothen blending from black to dark gray.
g2d.translate((win_w - img_w) / 2, win_h / 2 - img_h); g2d.drawImage(image, 0, 0, null);
The normal prototype is moved to the center of the window and drawn.
g2d.translate(0, 2 * imageHeight + gap); g2d.scale(1, -1);
This lawmaking flips the prototype and translates it beneath the original image. The translation performance is necessary because the scaling operation makes the image upside down and translates the prototype up. To understand what happens, simply take a photo and identify it on the tabular array and flip it.
g2d.drawImage(refImage, 0, 0, nada);
The reflected image is drawn.
@Override public Dimension getPreferredSize() { render new Dimension(img_w + 2 * SPACE, 2 * img_h + iii * Space); }
Another way to set a preferred size for a component is to override the getPreferredSize()
method.
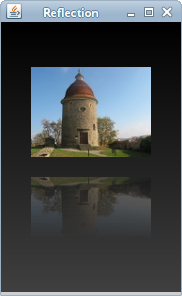
In this part of the Java2D tutorial we have worked with images.
Source: https://zetcode.com/gfx/java2d/java2dimages/
Posted by: singhhows2000.blogspot.com
0 Response to "How To Draw A Image In Java"
Post a Comment